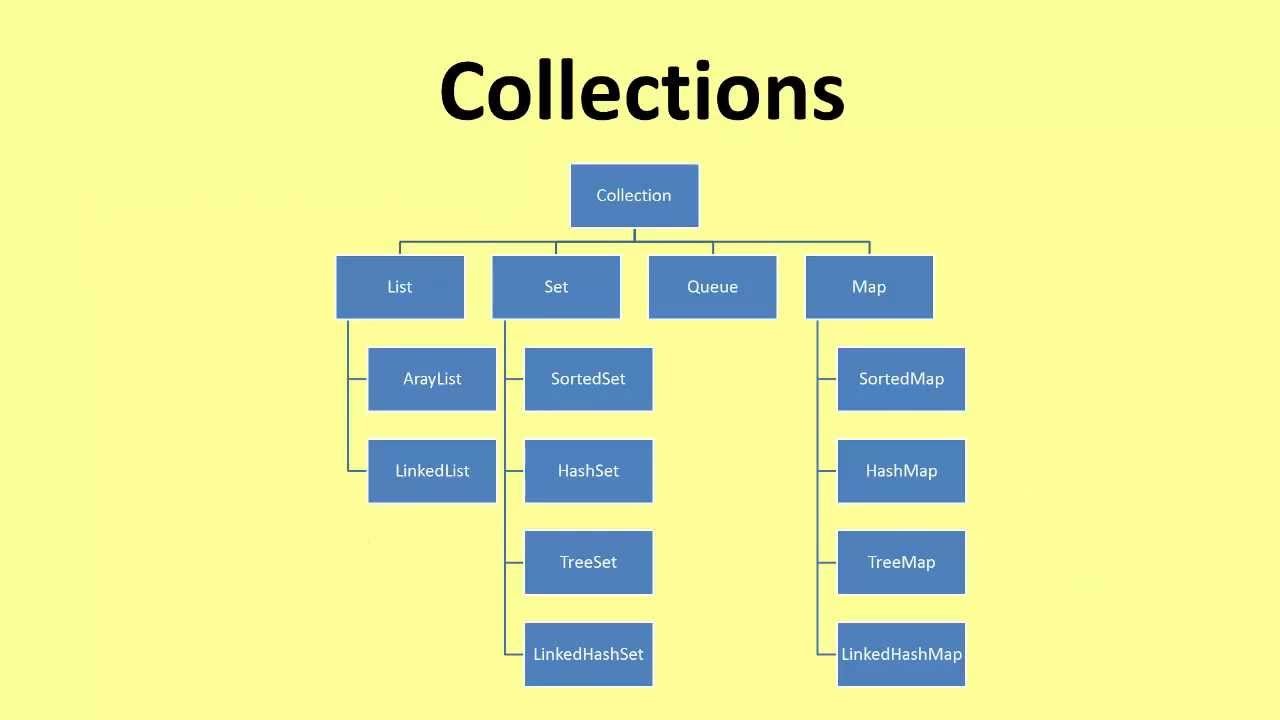
Understanding Java Data Structures
When diving into the world of programming, particularly in Java, one cannot overlook the significance of data structures. Java data structures are essential components that help in organizing, managing, and storing data efficiently. By mastering these structures, you’ll not only enhance your coding skills but also improve the performance of your applications. In this article, we’ll explore the various types of data structures in Java, their characteristics, and when to use them.
Table of Contents
ToggleWhat Are Data Structures?
Data structures are specialized formats for organizing, processing, and storing data. They allow programmers to manage large amounts of data efficiently and perform operations like searching, sorting, and manipulating data. In Java, data structures can be categorized mainly into two types: primitive and non-primitive.
Primitive Data Structures
Primitive data structures are the basic building blocks of data management in Java. They include:
- Integers: Whole numbers without decimal points.
- Floats: Numbers that can contain decimal points.
- Characters: Single letters or symbols.
- Booleans: True or false values.
Non-Primitive Data Structures
Non-primitive data structures are more complex and can store multiple values or a collection of data. They include:
- Arrays
- Linked Lists
- Stacks
- Queues
- Trees
- Graphs
These structures are foundational for building more complex systems, and understanding them is key for any aspiring Java programmer.
The Importance of Java Data Structures
When it comes to programming, the right choice of data structure can drastically affect the performance and efficiency of an application. Here are some reasons why understanding Java data structures is crucial:
- Efficiency: Different structures have varying speeds for insertion, deletion, and access. Selecting the right one can optimize performance.
- Memory Management: Understanding how data is stored allows for better memory utilization, reducing wastage and improving application performance.
- Data Manipulation: Certain structures are better suited for specific tasks, such as searching or sorting. Knowing which to use can simplify code and enhance functionality.
Common Java Data Structures
Let’s explore some of the most widely used Java data structures and their characteristics.
Arrays
Arrays are collections of elements, all of the same type, stored in contiguous memory locations. They are a fundamental data structure in Java.
Characteristics:
- Fixed size: The size must be defined at the time of creation.
- Indexed: Each element can be accessed via an index.
Usage:
- Storing a collection of items of the same type.
- Implementing other data structures like stacks and queues.
Example:
javaCopy codeint[] numbers = {1, 2, 3, 4, 5};
Linked Lists
A linked list is a linear data structure where each element (node) points to the next, allowing for dynamic memory allocation.
Characteristics:
- Dynamic size: Can grow and shrink during runtime.
- Non-contiguous memory allocation.
Types of Linked Lists:
- Singly Linked Lists: Each node points to the next node.
- Doubly Linked Lists: Each node points to both the next and previous nodes.
- Circular Linked Lists: The last node points back to the first.
Usage:
- Implementing stacks and queues.
- Dynamic memory allocation.
Example:
javaCopy codeclass Node {
int data;
Node next;
}
Stacks
A stack is a linear data structure that follows the Last In First Out (LIFO) principle. Think of it as a stack of plates; the last plate placed on top is the first one to be removed.
Characteristics:
- Push and pop operations.
- Can be implemented using arrays or linked lists.
Usage:
- Backtracking algorithms (like navigating browser history).
- Function calls in programming.
Example:
javaCopy codeStack<Integer> stack = new Stack<>();
stack.push(1);
stack.push(2);
int top = stack.pop(); // top is now 2
Queues
A queue is a linear data structure that follows the First In First Out (FIFO) principle. It’s like a line at a grocery store where the first person in line is the first to be served.
Characteristics:
- Enqueue (add) and dequeue (remove) operations.
- Can also be implemented using arrays or linked lists.
Usage:
- Managing requests in a server.
- Scheduling processes in operating systems.
Example:
javaCopy codeQueue<Integer> queue = new LinkedList<>();
queue.add(1);
queue.add(2);
int first = queue.poll(); // first is now 1
Trees
Trees are hierarchical data structures with a root node and child nodes, resembling an upside-down tree. They are crucial for organizing data in a way that makes searching and retrieval efficient.
Characteristics:
- Each node has a value and links to child nodes.
- Can be binary (each node has at most two children) or n-ary.
Types of Trees:
- Binary Trees: Each node has at most two children.
- Binary Search Trees (BST): Left child nodes are smaller, right child nodes are larger than the parent node.
- Balanced Trees: Trees that maintain a balanced height for efficient operations.
Usage:
- Storing hierarchical data (like file systems).
- Implementing searching algorithms.
Example:
javaCopy codeclass TreeNode {
int value;
TreeNode left;
TreeNode right;
}
Graphs
Graphs are non-linear data structures comprising nodes (vertices) and edges (connections between nodes). They can represent complex relationships and are used in various applications.
Characteristics:
- Can be directed (edges have a direction) or undirected.
- Can be weighted (edges have weights) or unweighted.
Usage:
- Social networks (friends and connections).
- Pathfinding algorithms (like GPS navigation).
Example:
javaCopy codeclass Graph {
Map<Integer, List<Integer>> adjacencyList = new HashMap<>();
}
Choosing the Right Data Structure
Selecting the appropriate Java data structures for a specific task can be a game changer. Here’s a quick guide:
Data Structure | Use Case | Characteristics |
---|---|---|
Array | Fixed-size collections | Contiguous memory, indexed access |
Linked List | Dynamic collections | Dynamic size, non-contiguous memory |
Stack | Undo mechanisms, function calls | LIFO principle, push/pop operations |
Queue | Task scheduling, printer jobs | FIFO principle, enqueue/dequeue operations |
Tree | Hierarchical data representation | Parent-child relationships, efficient searching |
Graph | Complex relationships, networks | Nodes and edges, can be directed or undirected |
Conclusion
Understanding Java data structures is a fundamental skill for any programmer. From organizing data efficiently to optimizing application performance, the right data structure can make a world of difference. With this guide, you now have a solid foundation to explore and utilize various data structures in your Java programming journey.
Key Takeaways
- Java data structures are essential for efficient data management.
- Different structures serve different purposes, so choose wisely based on your needs.
- Mastery of data structures will enhance your programming skills and application performance.